Format Strings in Python
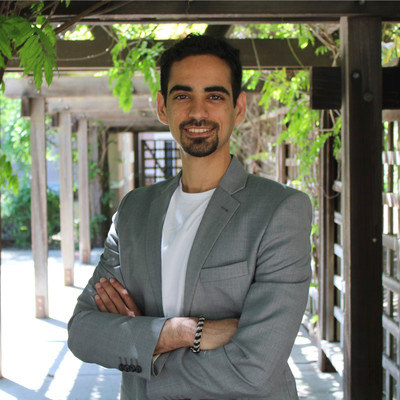
Ali Ismail
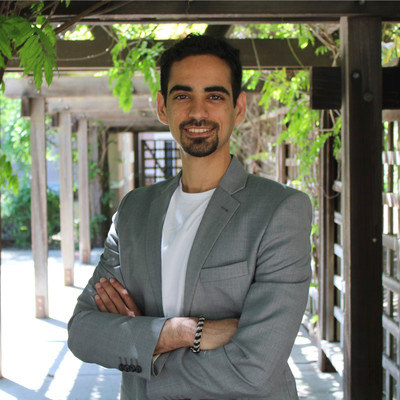
Ali Ismail
Format Strings in Python
Single Quotes
You can assign strings using single quotes in python
name = 'Ali'
Double Quotes
You can assign string using double quotes as well
name2 = "Ismail"
Triple Quotes
You can also assign strings that are multiline like so
name2 = """My Name is
Ail
Ismail"""
Note that the new line characters will be preserved when you print the values back on the screen
My Name is\nAli\nIsmail
Escaping Single Quotes
You can escape the ' character by using a \
print('Ali'\s test string')
This will read as
Ali's test string
Escaping Tabs
print('My\tfirst\tname\tis\tAli')
This will read as
My first name is ali
Substition Character
Let's assign First and Last Name and print it with the substitution character '%s'
first = "Ali"
last = "Ismail"
print("My name is %s %s" % (first, last))
String Format Operator
Another way to do this is by using the string format operator
first = "Ali"
last = "Ismail"
print("My name is {0} {1}}".format(first, last))
The number in the curly brackets tells the python interpretor which position in the variables to use.